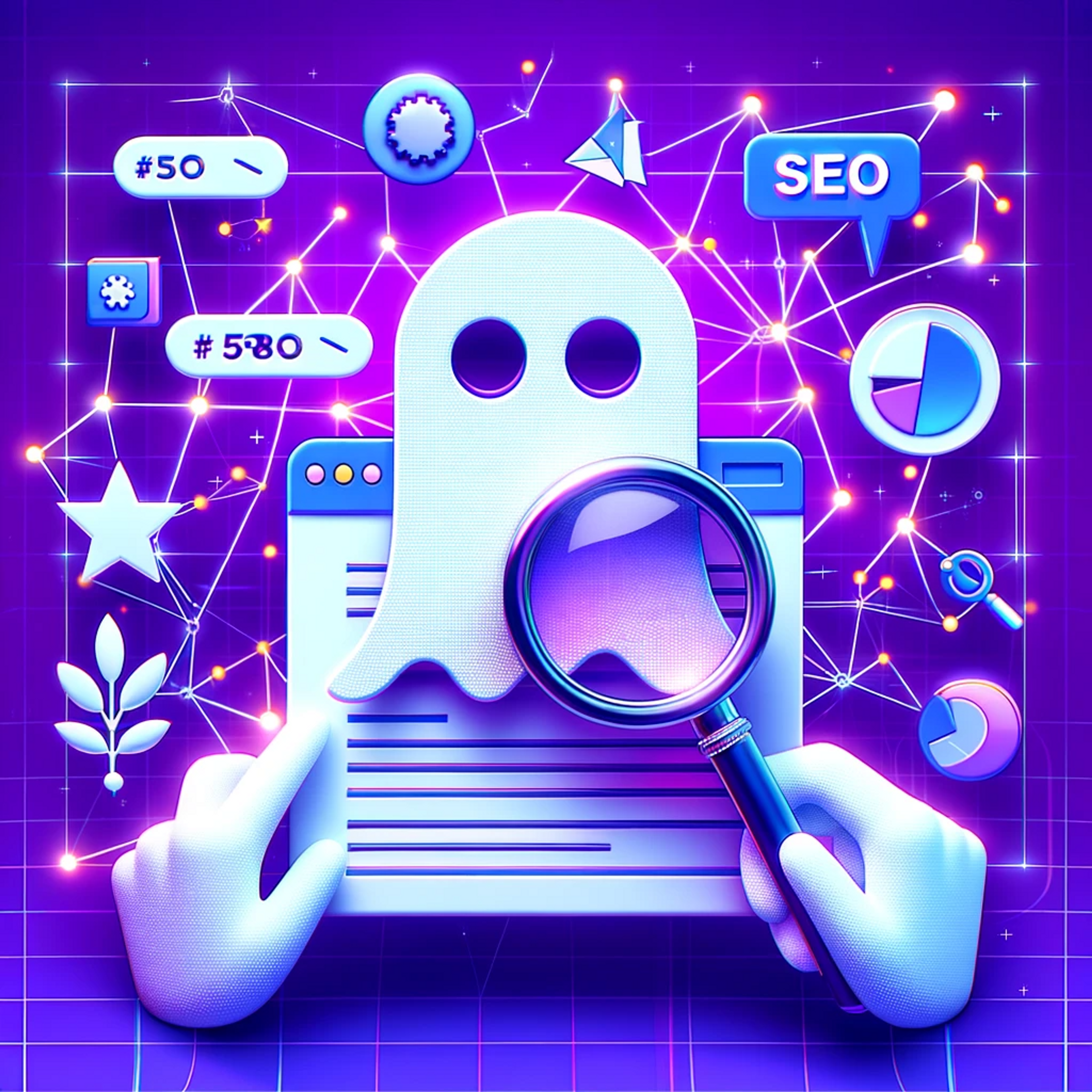
SEO with Next.js's Pages Router
- Next.js
- SEO
Contents
Search Engine Optimization (SEO) is crucial for ensuring that your website ranks well on search engines. In Next.js pages router, managing the metadata for your website can be streamlined with a reusable Meta component. Let's dive into how you can create a Meta component to handle all your SEO needs.
Creating a Reusable SEO Meta Component in Next.js
Every good SEO strategy begins with well-defined meta tags. With Next.js, this becomes easier to manage using the Head
component from next/head
.
Here's how you can create a reusable Meta
component and begin with adding the title and description of the page:
meta.tsx
1import Head from 'next/head';23interface Props {4 seo: {5 title: string;6 description: string;7 };8};910export default function Meta({ seo }: Props) {11 return (12 <Head>13 <title>{seo.title} | Stelko</title>14 <meta key="description" name="description" content={seo.description} />15 </Head>16 );17};
From there we can add our icons, manifest, and colours:
meta.tsx
1export default function Meta({ seo }: Props) {2 return (3 <Head>4 {/* Other meta tags */}5 <link6 rel="apple-touch-icon"7 sizes="180x180"8 href="/favicon/apple-touch-icon.png"9 />10 <link11 rel="icon"12 type="image/png"13 sizes="32x32"14 href="/favicon/favicon-32x32.png"15 />16 <link17 rel="icon"18 type="image/png"19 sizes="16x16"20 href="/favicon/favicon-16x16.png"21 />22 <link rel="manifest" href="/favicon/site.webmanifest" />23 <link rel="icon" href="/favicon/favicon.ico" />24 <meta name="msapplication-TileColor" content="#590bad" />25 <meta name="theme-color" content="#590bad" />26 </Head>27 );28};
To get your favicons and manifest created, you can go to https://favicon.io/ and convert your PNG logo to a ICO file. In return you will get a ZIP file containing all of your icons and manifest ready to be imported to your public folder. Just remember to edit your manifest to contain your info.
The last meta tag I will be adding is the canonical tag:
meta.tsx
1type Props {2 seo: {3 /* Other properties */4 slug: string;5 };6};78export default function Meta({ seo }: Props) {9 return (10 <Head>11 {/* Other meta tags */}12 <link13 rel="canonical"14 href={`https://stelko.xyz/${seo.slug}`}15 key="canonical"16 />17 </Head>18 );19};
Implementing the Meta Component on a Page
Now, let's implement the Meta
component in a page file:
index.tsx
1import Meta from '../components/Meta';23export default function Index() {4 return (5 <>6 <Meta7 title="London Next.js & Sanity Dev"8 description="Showcasing my expertise in Next.js and Sanity for dynamic, tailored web solutions. Let's bring your business to the forefront of the digital realm."9 slug=""10 />11 {/* Page content */}12 </>13 );14};
By importing and using the Meta
component, you can easily customize the meta tags for each page.
Dynamic Meta Tags for SEO
Dynamic pages in Next.js can benefit from dynamic meta tags. Suppose you have a blog post page where the meta tags depend on the post data:
[slug].tsx
1import { GetServerSideProps } from 'next';23import Meta from '../components/Meta';45interface Props {6 title: string;7 description: string;8 slug: string;9}1011export default function PostPage({ title, description, slug }: Props) => {12 return (13 <>14 <Meta title={title} description={description} slug={slug} />15 {/* Post content */}16 </>17 );18};1920export const getServerSideProps: GetServerSideProps = async (context) => {21 // Fetch post data based on id from the context22 // For illustration, we'll use static data23 const postData = {24 title: "Dynamic Page Title",25 description: "A description of the dynamic post.",26 slug: "post"27 };28 return { props: postData };29};
Conclusion
Good SEO practices are pivotal for the success of your website, and with Next.js, managing SEO with meta tags becomes a structured and efficient process. By creating a reusable Meta component and leveraging the power of server-side rendering for dynamic pages, you can ensure that your website stands out in search engine results.
Similar posts
- Modern web
- Sanity
- Next.js
- SEO
- Development
Generating a Sitemap with Next.js Pages Router and SanityJanuary 29, 2024
- Next.js
- Sanity
- SEO
SEO Magic with Next.js Pages Router and SanityNovember 20, 2023
- SEO
- Modern web
Mastering SEO Meta Title Tags: A Guide for Small BusinessesJanuary 08, 2024
- Modern web
- SEO
What is the Role of a Sitemap in Modern Web Design?January 01, 2024